- Chas McGill
- Oct 29, 2010
-
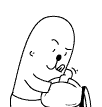
loves Fat Philippe
|
I'm currently learning Java as part of a degree on the Open University in the UK. It's my first programming language (Sense, an OU variant of Scratch, doesn't count) and I'm coming late to it.
While I'm enjoying the course, there's a load of course-specific terminology (messages instead of methods etc) and I'm concerned that I'm learning Java in a weird way. Can anyone recommend a definitive beginners guide to Java and OOP programming that reflects how the language is used in real applications?
|
#
¿
Jan 15, 2014 15:58
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
Apr 27, 2024 07:15
|
|
- Chas McGill
- Oct 29, 2010
-
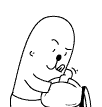
loves Fat Philippe
|
Have you seen the Oracle Java tutorials yet?
I've used them a bit when I've tried to explore concepts outside what the course has covered, but I'd prefer a paper book so I can study offline.
There's probably a way of turning them into PDFs or something, though.
|
#
¿
Jan 20, 2014 10:50
|
|
- Chas McGill
- Oct 29, 2010
-
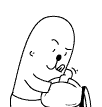
loves Fat Philippe
|
I'm really confused about how to show a sequence in a UML diagram where an object is returning results from one of its instance variables without using any methods to get those results. That probably isn't the best explanation, so here's a bit of code:
code: Map<Key, Value> getKeysAndValues()
{
Map<Key, Value> results = new HashMap<Key, Value>();
for (Key aKey : aCollection) //A collection held by the method's class.
{
results.put(aKey, aKey.getAValue());
}
return results;
}
Should I just show the keys and values returned by this process, or is there a way to represent the creation of the 'results' HashMap showing the keys returning their associated values?
I'm still learning Java and UML and I'm finding it tough to represent processes in the latter.
Here are three diagrams I've tried:

^Pretty sure this isn't right. It seemed like a good way to represent things until I actually drew it out.

^This is simplified, just showing the keys and values being accessed by the class. It still doesn't look right to me due to the lack of messages to those objects.

^This is the simplest and looks the most like other UML diagrams I've seen, but I also don't think it shows the process very well.
Any ideas are appreciated. I got myself in a bit of tizzy trying to figure this out earlier and I'm probably overthinking it massively.
|
#
¿
Feb 25, 2014 00:11
|
|
- Chas McGill
- Oct 29, 2010
-
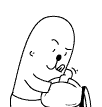
loves Fat Philippe
|
Is Swing worth checking out or is there a better alternative for simple UI stuff? I've been making a text based game as a project and I'd like to move beyond the console so I can have buttons to click on etc. I'm not planning on adding actual graphics or animation at the moment.
Alternatively, I'm also thinking of trying out Eclipse and the Android dev stuff, which I assume has its own UI bits that I could use.
Chas McGill fucked around with this message at 10:21 on Apr 8, 2014
|
#
¿
Apr 8, 2014 10:10
|
|
- Chas McGill
- Oct 29, 2010
-
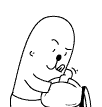
loves Fat Philippe
|
What's a good resource on learning how exceptions are to be used and added? I'm going to read through Oracle's lessons about them but am thinking that something meatier would be helpful to.
Seconding this. There's something about exception handling that just causes my brain to switch off when I'm trying to learn about it. I get confused by the exceptions hierarchy and when to use them instead of 'defensive programming'.
|
#
¿
Apr 11, 2014 13:16
|
|
- Chas McGill
- Oct 29, 2010
-
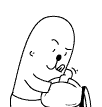
loves Fat Philippe
|
Exceptions are a "something wrong!" ...
Thanks very much for this, it's a much better explanation than the whole section in the textbook.
|
#
¿
Apr 14, 2014 17:42
|
|
- Chas McGill
- Oct 29, 2010
-
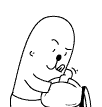
loves Fat Philippe
|
I've been banging my head against this all afternoon.
code:private int getNumber()
{
String numberString;
int number = 0;
boolean again = true;
while (again)
{
try
{
numberString = OUDialog.request("Enter a number between 1 and 10.");
number = Integer.parseInt(numberString);
if ((number < 1) || (number > 10))
{
OUDialog.alert("Entered value is outside range (between 1 and 10 inclusive)");
}
else
{
again = false;
}
}
catch (NumberFormatException numException)
{
OUDialog.alert("Input could not be converted to an integer. Please use a numeric value.");
}
}
return number;
}
OUDialog pops up with a dialog box asking for a String input. It returns null if the user hits cancel or closes it. My problem is that this causes the NumberFormatException to be caught, since a null value is an invalid input. It traps the user in a loop until they input the correct number, which isn't ideal. How do I separate the null value given by cancellation or closure from them inputting something like "bananas"? I've broken loops like this before by doing stuff like:
code:if (numberString == null)
{
again = false;
}
Chas McGill fucked around with this message at 14:58 on Apr 21, 2014
|
#
¿
Apr 21, 2014 14:54
|
|
- Chas McGill
- Oct 29, 2010
-
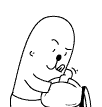
loves Fat Philippe
|
Edit: misread.
Check the return for null yourself? Like what you just edited in. Consider something closer to:
code:/** Returns a number, or null if cancelled */
private Integer getNumber()
{
while (true)
{
String numberString = OUDialog.request("Enter a number between 1 and 10.");
if(numberString == null) {
return null;
}
try
{
int number = Integer.parseInt(numberString);
if ((number >= 1) || (number <= 10))
{
return number;
}
OUDialog("Pick a number from 1 to 10");
}
catch (NumberFormatException numException)
{
OUDialog.alert("Input could not be converted to an integer. Please use a numeric value.");
}
}
}
Forgive typos, on phone.
drat, it'd take me hours to type code on my phone. That solution makes sense. I was definitely overthinking things - I've been learning about exceptions and was trying to think of a way to do everything using them - this works a lot better. Thanks.
|
#
¿
Apr 21, 2014 15:22
|
|
- Chas McGill
- Oct 29, 2010
-
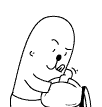
loves Fat Philippe
|
Arguably, you could make the method throw a checked exception instead of returning null, but that's probably not appropriate for this case. Exceptions are meant for exceptional behavior, not flow control.
Since I have my method returning an int, I can't return null, but I can get the 'effect' of cancel doing nothing by having it return 0 in that first if block. The other method I have is then passed that value of 0, which causes it to do...nothing.
This seems like bad programming though, so I've changed it to simply set 'again' to false, which breaks the loop and causes the NumberFormatException to display once. Not perfect, but at least the user can actually cancel out and they'll know why the other method hasn't continued.
Chas McGill fucked around with this message at 13:21 on Apr 23, 2014
|
#
¿
Apr 23, 2014 13:14
|
|
- Chas McGill
- Oct 29, 2010
-
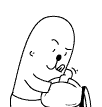
loves Fat Philippe
|
That's why his version returns an Integer, not an int. Integer is a class which wraps int and can be null. Since they're so darn similar, java will even automatically convert between Integer and int for you, which is known as autoboxing. It just means that
Java code:int x = 10;
Integer yInt = (Integer)x; //explicit class cast, valid
Integer xInt = x; //autoboxed, no explicit cast, also valid
int y = yInt; //autoboxed the other direction, valid
So you could switch your current code to use Integer and notice very little change. Be careful though that when you autobox to int if the value is null it will throw an exception. But your calling method can do a null check and then autobox to int, or you can just treat the result as an Integer.
But if 0 isn't a valid result, then you can use 0 or -1 or whatever as a 'flag' that the result was no good. Then you can just have a if(answer == -1) and if so don't call the next method and print error or whatever instead, and only call the next method in an else block.
Breaking the loop is okay, but is that the behavior you want? Wouldn't you prefer to ask the user for another input? Maybe I'm misunderstanding the problem.
Ah yeah, I've used Integers a bit in collections. I thought I was keeping it simple by using primitives, but I can see how that simplicity can lead to more work due to their inflexibility.
At the moment the loop causes the dialogue box display an alert and then reappear if the user enters a number outside the range. That's fine in the case of an incorrect input. What I was after is the 'cancel' behaviour you see in most applications - hit cancel and the box closes without anything else happening. I think your idea of handling an invalid return in the calling method is the best way to do it.
The way I had things set up previously was that getNumber() would return 0 (an invalid number) to the calling method, which would then take that 0 and perform its action 0 times. That seems a bit superfluous, even if it's getting me the result I want.
So I could instead do?:
code:public void callingMethod()
{
if (this.getNumber() == 0)
{
return;
}
else...
{
//method body
}
{
Java is my first programming language and it isn't coming easily to me.
Chas McGill fucked around with this message at 18:24 on Apr 23, 2014
|
#
¿
Apr 23, 2014 18:20
|
|
- Chas McGill
- Oct 29, 2010
-
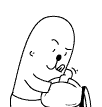
loves Fat Philippe
|
code: private Map<String, List<Book>> authorMap;
private List<Book> bibliography = new ArrayList<>();
public AuthorMap()
{
Map<String, List<Book>> authorMap = new HashMap<String, ArrayList<Book>>(); //incompatible type error
List<Book> bibliography = new ArrayList<>();
}
Why is this giving me an error for the commented part saying that I've got incompatible types when the instance variable declaration does the same thing (List<> -- ArrayList<>) and works?
I'm trying to create a map with a String as a key and and ArrayList of Book objects as a value. It is a complete ballache.
|
#
¿
Apr 25, 2014 16:08
|
|
- Chas McGill
- Oct 29, 2010
-
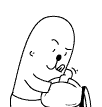
loves Fat Philippe
|
Variance is strange in Java. You have to explicitly say that the value in your map can be a List<Book> or any subtype of List<Book>.
Java code:Map<String, ? extends List<Book>> authorMap = new HashMap<String, ArrayList<Book>>()
Ugh, right. I'll just go with ArrayList for everything in that case, but I thought it was good practice to use a more generic interface in case things change later on - ie: using Map and then HashMap.
I think I've figured this out, although I'm concerned that my code is shite:
code:public class AuthorMap
{
private Map<String, ArrayList<Book>> authorMap;
private ArrayList<Book> bibliography = new ArrayList<>();
public AuthorMap()
{
authorMap = new HashMap<String, ArrayList<Book>>();
bibliography = new ArrayList<Book>();
}
public void printMap()
{
String newLine = System.getProperty("line.separator");
System.out.println("A list of the books by " + authorMap.keySet() + ":");
for (Book eachBook : bibliography)
{
System.out.println(newLine +
"Title: " + eachBook.getTitle() + newLine +
"Pages: " + eachBook.getLength() + newLine +
"Review Rating: " + eachBook.getRating() + newLine +
"Genre: " + eachBook.getGenre());
}
}
public void populateMap()
{
Book b1 = new Book("Author1", "Book1", 240, 4.5, "Genre1");
Book b2 = new Book("Author1", "Book2", 323, 3.3, "Genre1");
bibliography.add(b1);
bibliography.add(b2);
authorMap.put("Author1", bibliography);
}
Is this the best way to iterate through items of an ArrayList as a value in a map?
Output =
code:A list of the books by [Author1]:
Title: Book1
Pages: 240
Review Rating: 4.5
Genre: Genre1
Title: Book2
Pages: 323
Review Rating: 3.3
Genre: Genre1
Is there a reason you can't do:
HashMap<String, ArrayList<Book>> authorMap = new HashMap<String, ArrayList<Book>>(); ?
There's no reason I can't do that. I've just been in such a confused state today that it never occurred to me. I do usually head to Javadocs when I'm banging my head against a wall.
Chas McGill fucked around with this message at 16:40 on Apr 25, 2014
|
#
¿
Apr 25, 2014 16:31
|
|
- Chas McGill
- Oct 29, 2010
-
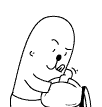
loves Fat Philippe
|
You're calling bibliography = new ArrayList<Book>(); twice, so get rid of one of them.
Otherwise that looks fine to me.
That's a perfectly good way to print an arrayList.
Just remember the limitation of the for-each loop ( for( : ) ) is that the collection you're iterating through can't change while you're doing so. For printing, this works great. But if you have to modify the list, you'll need to do something else, like a manual for( index = 0; ; ) or using an Iterator object and itr.next();
Also you're correct that leaving things generic or using interfaces can be good practice if things change down the line. But if you know what you want and especially since this is more for learning than writing production code, then you can just specify. Although its good to not learn bad habits! 
You could leave one as <> so it'll infer the type, or you could use ? extends List
Ah, good spot. I prefer to instantiate stuff in the constructor rather than when I declare the instance variable, so I'll delete the first one.
I started out intending to make a Map<String, String> and ended up thinking "well, wouldn't it be more interesting to have a list of stuff for each key?" and I've regretted it.
Thanks for your help.
|
#
¿
Apr 25, 2014 17:12
|
|
- Chas McGill
- Oct 29, 2010
-
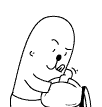
loves Fat Philippe
|
You are correct, but it's only *important* to do that when you're returning a collection (list/map/etc.), so that the caller can just treat it as a generic list/map/whatever, without worrying about whether it's an ArrayList or a LinkedList or some other thing.
In your case, rhag was correct. To reiterate:
Bad: Map<String, List<Book>> foo = new HashMap<String, ArrayList<Book>>()
Bad but working: Map<String, ArrayList<Book>> foo = new HashMap<String, ArrayList<Book>>()
Good: Map<String, List<Book>> foo = new HashMap<String, List<Book>>()
Better: Map<String, List<Book>> foo = new HashMap<>()
Let the compiler infer the entire right side for you.
Thanks. I'm now using the 'better' implementation except I've split the declaration, with the left side initialised as a private instance variable and the right side instantiating the variable in the constructor. It works fine and I don't get an incompatibility error. I was aware that the compiler can infer the type in blank <> - for some reason I decided to try and be specific. Of course, the more coding I have to do, the more errors I can make.
Now I'm trying to figure out how to add an arraylist for a specific key rather than running through all of them in the map. I did say that Java isn't coming easily, but I do tend to remember concepts once I've actually created code using them.
So, what you're wondering about is:
code:List<String> list1 = new ArrayList<String>(); // works
List<List<String>> list2 = new ArrayList<ArrayList<String>>(); // doesn't work
Because ArrayList<E> implements List<E> you get ArrayList<String> implements List<String> in the first version, which is why the assignment works. On the second line you get ArrayList<ArrayList<String>> implements List<ArrayList<String>> though.
This might not convince you, but think of the method List.add(E e). In one version this method would be add(List<String> e), but in the other it would be add(ArrayList<String> e), which are definitely not compatible!
code:List<List<String>> list = new ArrayList<ArrayList<String>>(); // this does not work because:
list.add(new LinkedList<String>()); // according tot the type of list, this should work, but
// since the list actually only accepts ArrayLists it would blow up.
That does make more sense to me now. I think previously I was just looking at the hierarchy and thinking "well, if I stick List on the left side it should accept whatever type that's under it" without thinking about the rest.
Chas McGill fucked around with this message at 14:04 on Apr 26, 2014
|
#
¿
Apr 26, 2014 13:55
|
|
- Chas McGill
- Oct 29, 2010
-
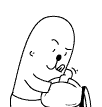
loves Fat Philippe
|
Can anyone recommend something similar to CodingBat for online, interactive Java problems? I like CodingBat a lot, but it doesn't cover a wide spread of the language. I have an exam next week and I find I tend to remember concepts more easily when I've actually coded something with them.
|
#
¿
May 27, 2014 10:40
|
|
- Chas McGill
- Oct 29, 2010
-
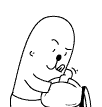
loves Fat Philippe
|
Got a question about composition vs inheritance.
Example:
An outdoor centre has bikes and boats available for rent.
Bike:
make
model
idNumber
ageGroup
Boat:
make
model
idNumber
capacity
Those are just examples of attributes the classes might have.
My inclination would be to have an abstract Vehicle class to capture their commonalities, but I've recently been learning about composition and also see its benefits. However, inheritance seems to 'make more sense' to me here since there's such an obvious is-a relationship. A Boat is a vehicle.
On the other hand, I feel like I'm probably ascribing too much real world meaning to the system. It feels weird to say that a boat references an instance of vehicle, rather than just being a vehicle. But it doesn't seem as weird to say that the role of Teacher has-a Person who fulfils it.
|
#
¿
Jun 26, 2014 14:07
|
|
- Chas McGill
- Oct 29, 2010
-
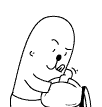
loves Fat Philippe
|
In your example inheritance seems like a fine solution. But you shouldn't think of composition as a boat referencing an instance of a vehicle. The boat is still a vehicle, it just maybe internally has an object that helps store some of its state like some sort of Model(make, model, id) class.
The value of composition comes from exposing partial functionality, or for example using dependency injection to easily swap out the guts of a class without anyone knowing about it.
I mean, the right answer is "whatever makes your code work." Do you have a situation where you have something that could be a boat or a bicycle, and you want to deal with whatever-it-is as a vehicle? Or do you have a Person, and want them to act as a Doctor in one situation, and a Mother in another? If you're not in either of these situations, gently caress it, don't bother making unnecessary hierarchical structures for the sake of having a hierarchy. It isn't terribly important that your code is an accurate description of The World, because you're not trying to model the world; you're usually trying to solve a very specific problem.
Thanks for the answers. I find structural stuff like this interesting, as well as the psychology of how the way I think code should work reflects how I think the world works, even if that doesn't necessarily translate to an efficient solution. It's tough to separate what a class symbolises to me and how it should function.
Going to read about dependency injection etc now.
It would be okay to use inheritance in this case, though most of the time, you use inheritance if you wish to inherit behavior, the code that's in the parent class.
In this case I'd probably have a RentableItem interface which includes all the stuff common to all rentable items that are needed to offer and rent an item (eg name, id, price, offer or pricelist reference, stuff like that) from the rental bookkeeping perspective, and leave the rest to evolve as you go for the time being. Interfaces are cool because they don't give dusty fucks about inheritance, any class can implement them, so you could as well have Tent or Boots classes with all kinds of different fields, but as long as they implement the methods dictated by the interface, from the sales perspective they're rentable assets all the same.
Yeah, I see how that would work as well. Composition and inheritance came up in the context of avoiding code duplication (in the course I'm doing) but having an interface seems like a more flexible solution for this kind of project.
Chas McGill fucked around with this message at 14:09 on Jun 27, 2014
|
#
¿
Jun 27, 2014 14:04
|
|
- Chas McGill
- Oct 29, 2010
-
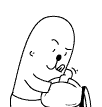
loves Fat Philippe
|
Thick question incoming:
How do I check if a collection only contains a certain value or object? I know about aCollection.contains(whatever) but I'm not sure how I'd make sure the collection doesn't contain anything else. I've been googling but haven't found anything useful.
(This is for a test method)
|
#
¿
Jul 2, 2014 13:56
|
|
- Chas McGill
- Oct 29, 2010
-
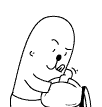
loves Fat Philippe
|
code:for (Object o : aCollection) {
if (o == null || !o.equals(whatever)) {
return false
}
}
return true;
Ugh what a dope I am. Thanks.
|
#
¿
Jul 2, 2014 14:26
|
|
- Chas McGill
- Oct 29, 2010
-
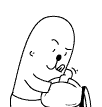
loves Fat Philippe
|
Either work for my purposes. Thanks. Having brain problems today. It's just for a test to make sure a collection only contains a specific object after a method is invoked.
|
#
¿
Jul 2, 2014 15:24
|
|
- Chas McGill
- Oct 29, 2010
-
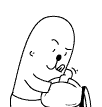
loves Fat Philippe
|
Java is my first programming language (not counting the Duplo that is Scratch) and I don't mind its verbosity either. I'd rather be able to read through a process on 4 lines and understand it than have it on 1 line made up of abbreviations and obscure symbols. I like the structure that the syntax forces at the moment. Maybe when I'm more experienced I'll chafe against it though.
|
#
¿
Jul 7, 2014 11:11
|
|
- Chas McGill
- Oct 29, 2010
-
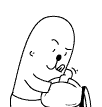
loves Fat Philippe
|
Guys I have a stupid question related to my previous question a few posts back.
Is there any way at all to override a field using field declarations in a subclass or is this something I have to do either by overriding the setter\getter methods or alternatively through defining a constructor in the super class?
i.e I have
code:Public class Snoop{
private int everyday = 420;
public int getEveryday(){
return this.everyday;}
}
and
code:Public class Dogg extends Snoop(
private int everyday = 840;
}
so Dogg.getEveryday() always returns 420 in this example, is there anyway to override the field in the declaration?
Thanks in advance
I don't know for sure, but you might have to override the getEveryDay() method to return the subclass field rather than using the inherited method.
code:public class Dogg extends Snoop
{
int everyDay = 840;
@Override
public int getEveryDay()
{
return this.everyDay;
}
}
Chas McGill fucked around with this message at 12:58 on Jul 14, 2014
|
#
¿
Jul 14, 2014 12:56
|
|
- Chas McGill
- Oct 29, 2010
-
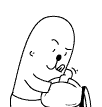
loves Fat Philippe
|
Following on from this - marketing at work wants to create a bit of modelling software to visualise the effect of spend on oil platforms. Basically it'd be a picture that changes when the user moves sliders or inputs figures. Are there any good Java libraries for something like this, or should I be looking at another tool/language? Seems like flash would be the traditional thing, but I only have (limited) experience with Java.
|
#
¿
Sep 3, 2014 08:58
|
|
- Chas McGill
- Oct 29, 2010
-
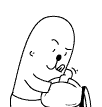
loves Fat Philippe
|
I'm trying to use the new LocalDate class in Java 8 and for some reason I'm getting an error in IntelliJ that its constructor is private, despite the javadoc listing it as public.
I expect I'm missing something fairly obvious here. I've imported the correct package. I just want a toString() friendly date format without any time information.
|
#
¿
Sep 26, 2014 14:09
|
|
- Chas McGill
- Oct 29, 2010
-
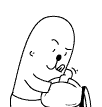
loves Fat Philippe
|
That's what I get for googling something and looking at the first result without thinking about it! I think I was still thinking in terms of the old Date class, which does have a public constructor.
I got the result I wanted with this:
LocalDate dob = LocalDate.of(1950,10,14);
Chas McGill fucked around with this message at 15:17 on Sep 26, 2014
|
#
¿
Sep 26, 2014 15:14
|
|
- Chas McGill
- Oct 29, 2010
-
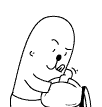
loves Fat Philippe
|
It's okay. At least you get to play with cool features. Work just crushes me by forcing me to stay in the bounds of Java 6 if I'm lucky.
Well, I just finished a couple of Java modules so this is just for a personal project to stop everything I learnt from leaking out of my brain now that they're over. I remember worrying that what I was being taught would be obsolete within a year, but that was before I understood how much legacy work there is.
|
#
¿
Sep 26, 2014 16:01
|
|
- Chas McGill
- Oct 29, 2010
-
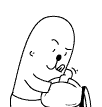
loves Fat Philippe
|
The modules are:
Object-oriented Java programming (M250)
This module concentrates on aspects of Java that best demonstrate object-oriented principles and good practice, you'll gain a solid basis for further study of the Java language and object-oriented software development.
Software development with Java (M256)
Discover the fundamentals of an object-oriented approach to software development, using up-to-date analytical techniques and processes essential for specification, design and implementation.
Mate, I just did those two modules. Don't worry too much about it. If you have any programming experience you'll be in a better position than I was to start with. M250 is baby's first java and M256 is jargon memorisation. Feel free to ask me any questions about the modules. I got a distinction for M250 and I'm anxiously waiting on the M256 results.
for (Frog eachFrog : frogTribe)
{
eachFrog.croak();
}
if (kermit.isGreen())
{
kermit.matingDance();
}
edit: Feels weird to see someone posting about the OU on here.
Chas McGill fucked around with this message at 23:55 on Sep 30, 2014
|
#
¿
Sep 30, 2014 23:50
|
|
- Chas McGill
- Oct 29, 2010
-
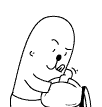
loves Fat Philippe
|
I started those at one time about 10 years ago, they started easy and then got mega hard even for a seasoned pro,
I hope you have lots of time as there was soooo much studying.
Although they keep saying that courses are being dumbed down.
The toughest thing about them is the amount of reading/memorisation required. M250 introduces almost every basic concept in Java (although it bizarrely omits switches and the ternary operator), M256 tries to be a primer on how to design software, but I had a tough time believing anyone develops in its style outside the NHS (probably). I found the TMAs fairly easy since I could refer to the materials.
Both exams were balls hard though.
|
#
¿
Oct 1, 2014 08:48
|
|
- Chas McGill
- Oct 29, 2010
-
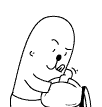
loves Fat Philippe
|
Looks like I am going to have fun then! I take it the exam is the vast majority of the grade too :/
Your final grade will be split between your continuous assessment (TMAs, ICMAs) and the exam. You need +85% in both components to get a distinction, but you only need 40% to pass.
The most advanced thing you'll do in M250 is probably reading from and writing to CSV files, or iterating through collections.
M256 has very little programming since it's mostly theoretical.
The course materials for both modules is extremely wordy, so if you're struggling with any concept you can probably just google it and find a much more concise explanation on stack overflow/youtube/javadocs.
I will say that I feel like M250 gave me a decent grounding in Java basics and M256 got me interested in design patterns etc.
|
#
¿
Oct 1, 2014 10:01
|
|
- Chas McGill
- Oct 29, 2010
-
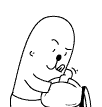
loves Fat Philippe
|
Is this a weird way to use exceptions (this is taken from a bit of homework I did earlier in the year):
code:public void doBorrowBook()
{
Member theMember = (Member) memberList.getSelectedValue();
Book theBook = (Book) booksToBorrowList.getSelectedValue();
try
{
Loan theLoan = library.borrowBook(theBook, theMember);
outcomeArea.setText(theLoan.toString());
}
catch (IllegalStateException stateException)
{
if (library.hasMaximumLoans(theMember))
{
outcomeArea.setText(stateException.getMessage()
+ System.getProperty("line.separator")
+ "A book must be returned before a new loan is permitted.");
/*
* This handles the case where the maximum number of loans has been reached.
* The exception message doesn't mention that a book has to be returned.
* Exception in borrowBook().
*/
}
else outcomeArea.setText(stateException.getMessage());
//This handles any other IllegalStateExceptions.
}
catch (IllegalArgumentException argException)
{
outcomeArea.setText(argException.getMessage());
/*
* This handles the case where the member already has the book on loan.
* displayAvailableBooks() defends against this eventuality in this screen,
* but it doesn't hurt to catch the exception since the system may change.
*/
}
}
I did it like that because I wanted to use the exception messages in the other class in the outcome area of the GUI. I didn't get marked down for it, but the tutor said it was unnecessarily complex.
|
#
¿
Oct 9, 2014 14:10
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
Apr 27, 2024 07:15
|
|
- Chas McGill
- Oct 29, 2010
-
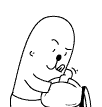
loves Fat Philippe
|
That's what people are complaining about. There's nothing exceptional about those exceptions. Check if the book's been loaned or whatever your preconditions are before you try to loan it to the member. It's fine in a small, one-off project, but it's a pain in the rear end to try and debug why poo poo's broke years down the line.
This. Don't rely on exceptions to be your flow control. Exceptions should be an uncommon, unexpected event that is still possible to occur. Think IOExceptions for an unexpected network disconnection.
Yeah, I won't do it again!
|
#
¿
Oct 10, 2014 09:31
|
|