- Yakattak
- Dec 17, 2009
-
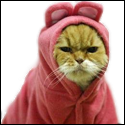
I am Grumpypuss
>:3
|
I have a programming question for the Arduino. We have a keypad that is used to "dial a phone". We got the numbers working perfectly fine, when you hit 1, it means 1. The problem we're having is we can't get it to act like a phone, in the sense that, when you dial 1, and wait like a second or two, it will go and dial that number, but when you hit 1,2 and 3 within that period, it dials 123. Is this possible at all?
code:#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 3; //three columns
char keys[ROWS][COLS] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'#','0','*'}
};
byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup(){
Serial.begin(9600);
}
void loop(){
char key = keypad.getKey();
if (key != NO_KEY){
Serial.println(key);
}
}
So, I'd change "key" to an array, and have that array of numbers print instead.
Yakattak fucked around with this message at 15:42 on Mar 10, 2013
|
#
¿
Mar 10, 2013 15:40
|
|
- Adbot
-
ADBOT LOVES YOU
|
|
#
¿
May 3, 2024 09:13
|
|
- Yakattak
- Dec 17, 2009
-
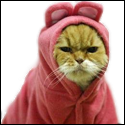
I am Grumpypuss
>:3
|
That is totally possible. Easiest way is probably to use the Metro library as a timer to see if ~200 ms has passed between keypresses. I haven't used it myself so this may not be right, but here's something along the lines of what you want:
code:Metro keypressTimer = Metro(2000); // set up timer with 2000ms period
char keyArray[10] = {'\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0'};
int keyArrayIndex = 0;
void loop(){
if( keypressTimer.check() == 1 && keyArray[0] != '\0' ) { // if timer expired and keyArray has contents
Serial.println(keyArray); // print keyArray
keyArray = {'\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0', '\0'}; // clear keyArray
keyArrayIndex = 0; // reset keyArrayIndex
}
char key = keypad.getKey(); // read in key press
if (key != NO_KEY){ // if we got a value for the key press
keyArray[keyArrayIndex] = key; // put key press into keyArray
if(keyArrayIndex < 9) {keyArrayIndex++}; // increment index if it won't overflow
keypressTimer.reset(); // reset timer
}
}
The above makes several assumptions. I haven't included most of your keypad code. The length of keyArray should be set to the longest string of characters you expect. The tenth input value will be modified if more keys are pressed before the timer expires but after you've filled the array. I am assuming that \0 is an acceptable way to write a null character. You might be better off using a string object rather than an array for this. I haven't tested this to see if it will run or even checked it very thoroughly for correctness. You can also roll your own timer using millis() instead of using Metro.
But that's a basic way you can do it. Run a timer which resets each time a key is pressed, and only output when the timer expires or reaches a certain value.
You are a god among men, thank you. Can't wait to try this out!
|
#
¿
Mar 10, 2013 21:43
|
|